I have not worked with excel files from C++, specifically, but github appears to have these 3 projects that are each quite popular.
OpenXLSX (The readme specifically metnions that it works with VS 2019 using the cmake extension)
Many programming languages have the ability to modify Excel files,
either natively or in the form of open source libraries. This includes
Python, Java and C#. For C++, however, things are more scattered.
While there are some libraries, they are generally less mature and
have a smaller feature set than for other languages.
Because there are no open source library that fully fitted my needs, I
decided to develop the OpenXLSX library.
xlnt appears to be the C++ Excel library with the most stars on github. It appears that its syntax closely matches Excel's own. Its feature list indicates that it supports a standard spreadsheet made up of simple column/row data, but I also see it specifically does not support "Tables", so you might want to double check if this one is going to work for you before diving in.
readxl claims to offer a simplicity which they suggest matches this cheatsheet from rstudio:
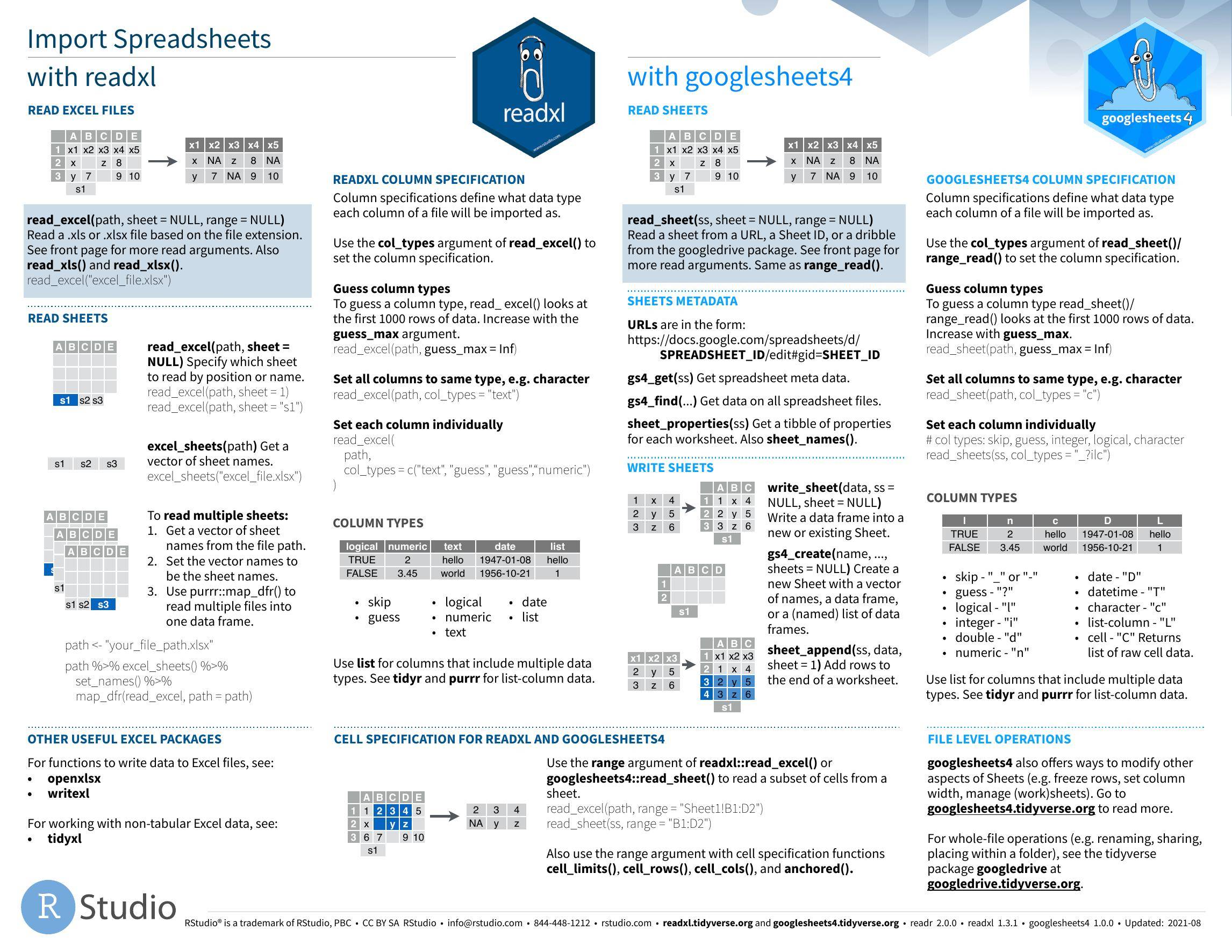
If you're curious, I found these using a wonderful new(to me) tool https://www.awesomeopensource.com that makes finding the gems hidden within github a breeze with its simple category based listings ranked by stars. I know that sounds like something easily done within github itself, but I implore you to give it a try and see the difference!